By default, WooCommerce allows your customers to change the quantity of a product in the cart. This is perfect for a lot of stores, but there are sometimes situations where you’ll want to prevent your customers from changing the quantity in the cart area.
This was a simple task when using the old shortcode, but if you’re using the new WooCommerce Cart Block, you’ll notice that our old snippet doesn’t work for that.
The WooCommerce Cart block is still pretty new, and doesn’t currently have a whole host of modifications available. However, there are still a snippet that you can use to prevent your customers from changing the quantity in the cart when using the WooCommerce Cart Block.
The snippet modifies the min/max quantity of the cart item, effectively preventing the customer from increasing or decreasing the quantity when on the cart. We can then hide the disabled +/- buttons using CSS.
First of all, we’ll look at the snippet to disable the quantity change.
Apply to All Products
This snippet will disable the WooCommerce cart quantity field for all products:
add_filter( 'woocommerce_store_api_product_quantity_minimum', 'snippetpress_disable_wc_cart_block_quantity', 10, 3 );
add_filter( 'woocommerce_store_api_product_quantity_maximum', 'snippetpress_disable_wc_cart_block_quantity', 10, 3 );
function snippetpress_disable_wc_cart_block_quantity( $value, $product, $cart_item ){
if( ! $cart_item ) return $value;
return $cart_item['quantity'];
}
Apply to Specific Products
This snippet will disable the WooCommerce cart quantity for specific products. Just add your product IDs in the $disable_qty
array on line 7:
add_filter( 'woocommerce_store_api_product_quantity_minimum', 'snippetpress_disable_wc_cart_block_quantity', 10, 3 );
add_filter( 'woocommerce_store_api_product_quantity_maximum', 'snippetpress_disable_wc_cart_block_quantity', 10, 3 );
function snippetpress_disable_wc_cart_block_quantity( $value, $product, $cart_item ){
if( ! $cart_item ) return $value;
$disable_qty = array(123, 456, 789); // Add your WC product IDs to the array
if ( in_array( $product->get_id(), $disable_qty ) ) {
return $cart_item['quantity'];
}
return $value;
}
Apply to all products except specific products
This snippet will allow you to disable the WooCommerce cart quantity field on all products except for the ones you specify. Just add the products that you want to exclude from the snippet to the $enable_qty
array on line 7:
add_filter( 'woocommerce_store_api_product_quantity_minimum', 'snippetpress_disable_wc_cart_block_quantity', 10, 3 );
add_filter( 'woocommerce_store_api_product_quantity_maximum', 'snippetpress_disable_wc_cart_block_quantity', 10, 3 );
function snippetpress_disable_wc_cart_block_quantity( $value, $product, $cart_item ){
if( ! $cart_item ) return $value;
$disable_qty = array(123, 456, 789); // Add your WC product IDs to the array
if ( !in_array( $product->get_id(), $disable_qty ) ) {
return $cart_item['quantity'];
}
return $value;
}
Hide the +/- buttons with CSS
Now that you’ve installed the snippet to disable the quantity change, you’ll see that clicking on the +/- buttons in the cart does nothing, and you can’t enter a new quantity into the field. However, the +/- buttons are still showing, which could be confusing for a customer. The good news is, as our snippet has set these buttons to disabled, we can target them using some CSS to hide them:
.wc-block-cart-item__quantity .wc-block-components-quantity-selector__button:disabled{
display: none;
}
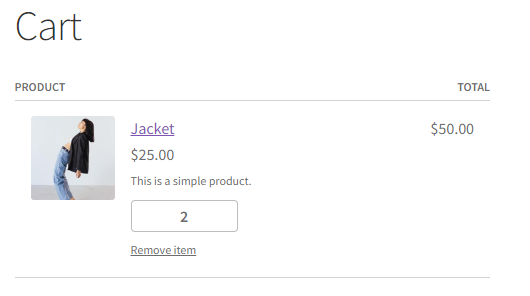
You can add this CSS in your theme’s Customizer. Once you’ve installed the CSS, the +/- buttons will be removed from the cart item.
Where to add the snippet?
Whichever snippet you choose to use, you should place it at the bottom of the functions.php file of your child theme. Make sure you know what you’re doing when editing this file. Alternatively, you can use a plugin such as Code Snippets to add the custom code to your WordPress site. If you need further guidance on how to add the code, check out our post on How to Add WordPress Snippets.